QMessageBox 은 사용자가 버튼을 클릭해서 요구한 내용에 대해 결과나 관련 정보를 통보하기 위해 쓰이는 대화상자입니다. 기본적으로 QMessageBox 를 종료하지 않으면 부모창을 제어할 수 없는 Model 대화상자입니다.
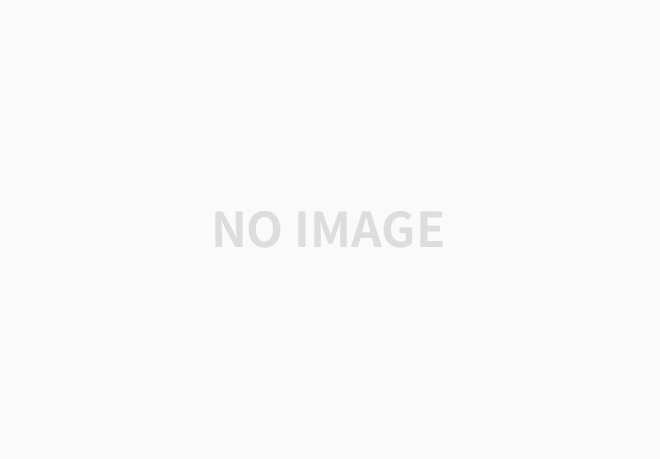
▼ 메시지박스는 QMessageBox 클래스를 사용합니다. 사용을 위해 QMessageBox 객체를 생성합니다. 클래스는 PyQt5.QtWidgets 패키지 안에 있습니다.
from PyQt5.QtWidgets import QMessageBox # QDialog 설정 self.msg = QMessageBox()
▼ 메시지박스 실행을 위해 버튼을 추가하고 Clicked 이벤트 슬롯에 messagebox_open() 사용자 함수를 추가했습니다. 내부에는 QMessageBox 객체 관련 설정들이 들어가 있습니다.
self.button.clicked.connect(self.messagebox_open) # 버튼 이벤트 함수 def messagebox_open(self): self.msg.setIcon(QMessageBox.Information) self.msg.setWindowTitle('MessageBox Test') self.msg.setText('MessageBox Information') self.msg.setStandardButtons(QMessageBox.Ok | QMessageBox.Cancel) retval = self.msg.exec_()
▼ MessageBox 설정은 다음과 같습니다.
self.msg.setIcon(QMessageBox.Information) self.msg.setWindowTitle('MessageBox Test') self.msg.setText('MessageBox Information') self.msg.setStandardButtons(QMessageBox.Ok | QMessageBox.Cancel) retval = self.msg.exec_()
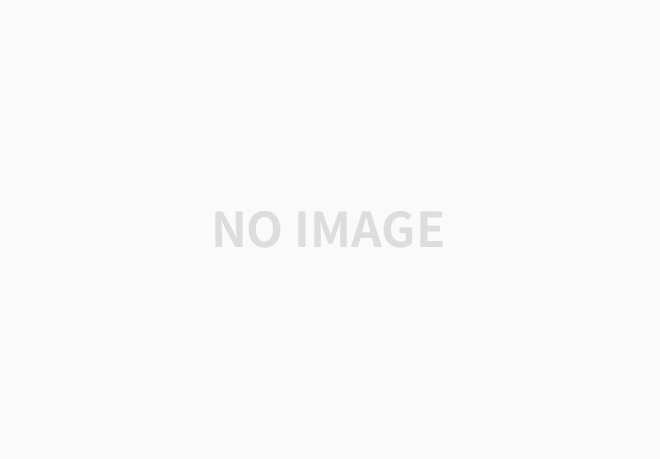
▼ MessageBox 의 setIcon() 은 화면에서 왼쪽 느낌표 아이콘을 말합니다. 종류는 다음과 같습니다.
l QMessageBox.NoIcon : 값은 0 이며, 기본값이다. 메시지 박스에 아이콘을 표시
l QMessageBox.Information : 값은 1 이며, 느낌표 아이콘 표시
l QMessageBox.Warning : 값은 2 이며, 느낌표 아이콘에 배경이 노란색 삼각형 표시
l QMessageBox.Critial : 값은 3 이며, 오류를 나타낼 때 표시
l QMessageBox.Question : 값은 4 이며, 물음표 아이콘 표시
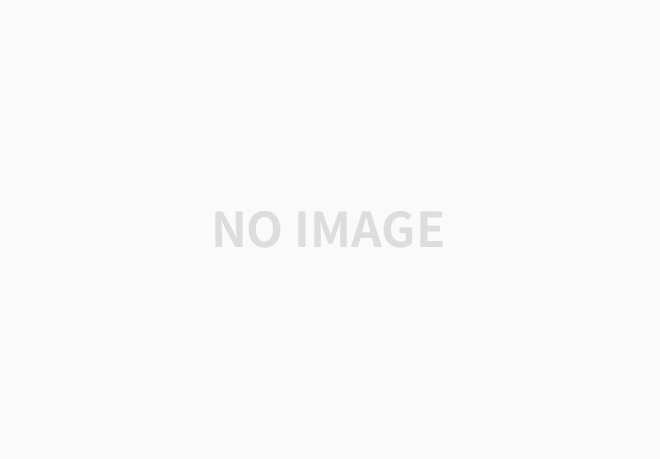
▼ setStandardButton() 함수의 파라미터로 아래 값들을 조합해서 입력합니다. 비트 연산자 중 OR(|) 을 사용해서 아래 두 가지 이상의 플래그를 조합니다.
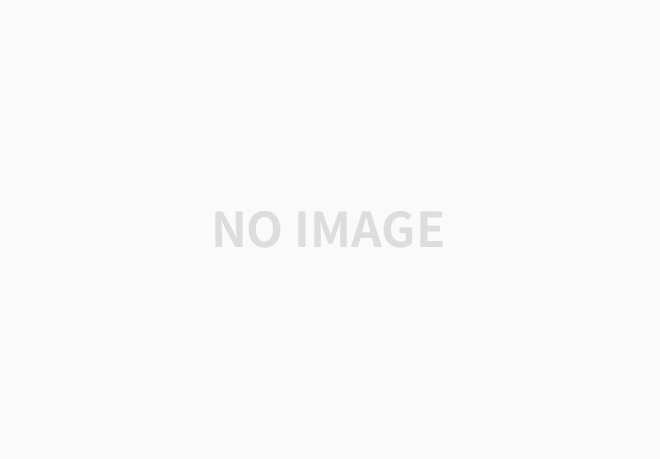
▼ 메시지박스에서 어느 버튼을 클릭했는지는 어떻게 판단할까요? QMessageBox 의 exec_() 함수를 실행하고 받은 리턴값을 QMessageBox 플래그로 비교하는 것입니다.
retval = self.msg.exec_() # 반환값 판단 print('QMessageBox 리턴값 ', retval) if retval == QMessageBox.Ok : print('messagebox ok : ', retval) elif retval == QMessageBox.Cancel : print('messagebox cancel : ', retval)
▼ 전체 소스는 다음과 같습니다.
#!/usr/bin/env python3 import sys from PyQt5.QtWidgets import * class MainWindow(QMainWindow): def __init__(self): super().__init__() # 윈도우 설정 self.setGeometry(300, 300, 400, 300) # x, y, w, h self.setWindowTitle('Status Window') # QButton 위젯 생성 self.button = QPushButton('MessageBox Button', self) self.button.clicked.connect(self.messagebox_open) self.button.setGeometry(10, 10, 200, 50) # QDialog 설정 self.msg = QMessageBox() # 버튼 이벤트 함수 def messagebox_open(self): self.msg.setIcon(QMessageBox.Information) self.msg.setWindowTitle('MessageBox Test') self.msg.setText('MessageBox Information') self.msg.setStandardButtons(QMessageBox.Ok | QMessageBox.Cancel) retval = self.msg.exec_() # 반환값 판단 print('QMessageBox 리턴값 ', retval) if retval == QMessageBox.Ok : print('messagebox ok : ', retval) elif retval == QMessageBox.Cancel : print('messagebox cancel : ', retval) if __name__ == '__main__': app = QApplication(sys.argv) mainWindow = MainWindow() mainWindow.show() sys.exit(app.exec_())
'파이썬 Python' 카테고리의 다른 글
파이썬(Python) 문자열을 나눌 수 있는 split 함수 (1) | 2024.07.21 |
---|---|
파이썬(Python) Matplotlib 설치 하고 샘플 구동하기 (0) | 2024.07.21 |
파이썬(Python) 두 개의 숫자 더하기 (0) | 2024.07.09 |
파이썬(Python) PIP freeze 설치 라이브러리 복구하기 (0) | 2024.07.09 |
파이썬(Python) PyQt5 QStatusBar 구현하기 (0) | 2024.07.07 |
파이썬(Python) type 함수 사용하기 (0) | 2024.07.06 |
파이썬(Python) PyQt5 QSlider 사용하기 (0) | 2024.06.30 |
파이썬(Python) 개발도구(PyCharm) 단축키 설정과 검색하는 방법 (2) | 2024.04.11 |