“ArrayList”를 초기화하는 방법은 여러 가지가 있습니다. 주로 생성자를 통한 초기화나 메서드를 이용한 초기화 등을 사용합니다. 생성자를 이용하거나 Arrays.asList() 와 Collections.addAll() 함수를 이용한 방법을 소개하겠습니다.
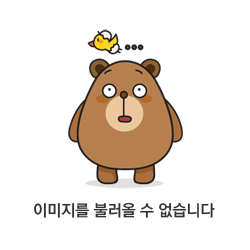
◎ 구문 |
1. 생성자를 이용한 초기화
아래 구문은 “ArrayList”를 생성하는 코드입니다. “ArrayList”는 크기를 동적으로 조절할 수 있는 배열 기반의 리스트입니다. “<Type>“은 리스트에 저장되는 요소들의 데이터 타입을 나타냅니다.
ArrayList<Type> list = new ArrayList<>(); |
구문은 초기 용량(“initialCapacity”)을 지정하여 “ArrayList”를 생성하는 코드입니다. “initialCapacity”는 리스트가 처음에 할당하는 용량을 나타내며, 이후 요소가 추가될 때 동적으로 용량이 조절됩니다.
ArrayList<Type> listWithCapacity = new ArrayList<>(initialCapacity); |
구문은 다른 “ArrayList”인 “anotherList”의 내용을 복사하여 새로운 “ArrayList”를 생성하는 코드입니다. 즉, “copyList”와 “anotherList”는 동일한 요소를 가지지만 서로 독립적인 인스턴스입니다.
ArrayList<Type> copyList = new ArrayList<>(anotherList); |
2. Arrays.asList() 메서드를 이용한 초기화
구문은 “Arrays.asList()” 메서드를 사용하여 주어진 요소들로 초기화된 “ArrayList”를 생성하는 코드입니다. 생성된 “ArrayList”는 “List<Type>“ 인터페이스로 참조합니다. “<Type>“은 리스트에 저장되는 요소들의 데이터 타입을 나타냅니다.
List<Type> list = new ArrayList<>(Arrays.asList(element1, element2, ...)); |
3. Collections.addAll() 메서드를 이용한 초기화
구문은 “Collections.addAll()” 메서드를 사용하여 주어진 요소들로 초기화된 “ArrayList”를 생성하는 코드입니다. 생성된 “ArrayList”는 “List<Type>“ 인터페이스로 참조됩니다. “<Type>“은 리스트에 저장되는 요소들의 데이터 타입을 나타냅니다.
List<Type> list = new ArrayList<>(); Collections.addAll(list, element1, element2, ...); |
◎ 예제 1: 생성자를 이용한 초기화 |
예제에서는 생성자를 이용하여 빈 “ArrayList”와 초기 용량이 있는 “ArrayList”, 그리고 다른 컬렉션에서 복사한 “ArrayList”를 생성했습니다.
import java.util.ArrayList; public class ArrayListInitializationExample { public static void main(String[] args) { // 빈 ArrayList 생성 ArrayList<String> emptyList = new ArrayList<>(); System.out.println("빈 ArrayList: " + emptyList); // 초기 용량을 지정한 ArrayList 생성 ArrayList<Integer> listWithCapacity = new ArrayList<>(10); System.out.println("초기 용량이 있는 ArrayList: " + listWithCapacity); // 다른 컬렉션의 요소로 초기화한 ArrayList 생성 ArrayList<String> sourceList = new ArrayList<>(); sourceList.add("apple"); sourceList.add("banana"); ArrayList<String> copyList = new ArrayList<>(sourceList); System.out.println("다른 컬렉션에서 복사한 ArrayList: " + copyList); } } [출력] 빈 ArrayList: [] 초기 용량이 있는 ArrayList: [] 다른 컬렉션에서 복사한 ArrayList: [apple, banana]
◎ 예제 2: Arrays.asList() 메서드를 이용한 초기화 |
예제에서는 “Arrays.asList()” 메서드를 사용하여 초기화한 “ArrayList”를 생성했습니다. 이 메서드는 가변 인자를 사용하여 배열이나 요소들을 리스트로 변환할 수 있습니다.
import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class ArrayListInitializationExample2 { public static void main(String[] args) { // Arrays.asList() 메서드를 이용한 초기화 List<String> fruits = new ArrayList<>(Arrays.asList("apple", "orange", "banana")); System.out.println("과일 목록: " + fruits); } } [출력] 과일 목록: [apple, orange, banana]
◎ 예제 3: Collections.addAll() 메서드를 이용한 초기화 |
예제에서는 “Collections.addAll()” 메서드를 사용하여 초기화한 “ArrayList”를 생성했습니다. 이 메서드는 가변 인자를 사용하여 컬렉션에 여러 요소를 추가할 수 있습니다.
import java.util.ArrayList; import java.util.Collections; public class ArrayListInitializationExample3 { public static void main(String[] args) { // Collections.addAll() 메서드를 이용한 초기화 ArrayList<Integer> numbers = new ArrayList<>(); Collections.addAll(numbers, 1, 2, 3, 4, 5); System.out.println("숫자 목록: " + numbers); } } [출력] 숫자 목록: [1, 2, 3, 4, 5]
'자바(JAVA)' 카테고리의 다른 글
자바(Java) String 배열 정렬하기 (1) | 2024.02.26 |
---|---|
자바(Java) replace() 와 replaceAll() 함수의 차이는? (0) | 2024.02.20 |
자바 OpenJDK 설치와 환경 변수 설정하기 (0) | 2024.02.18 |
자바(Java) String 배열 정렬하기 (0) | 2024.02.16 |
자바(Java) 문자열 공백 제거하는 방법 (trim, replaceAll) (0) | 2024.02.01 |
자바(Java) 주석 Comments 소스 내에 작성하는 방법 (2) | 2024.01.25 |
자바 Java 이클립스를 이용해서 자바 디버깅 하기 (10) | 2024.01.14 |
스프링 (Spring Boot) 메이븐 배포시 No Compiler is provided in this environment 에러 (7) | 2024.01.10 |
녹두장군1님의
글이 좋았다면 응원을 보내주세요!