프로그램 언어에서 가장 자주 찾는 항목이 날짜 계산입니다. 데이터베이스 테이블 설계할 때 날짜는 항상 들어가고 꺼내서 가공할 때도 날짜를 기준으로 많이 이용하기 때문입니다. 자바스크립트로 날짜를 계산하는 여러가지 방법들에 대해 알아봅니다.
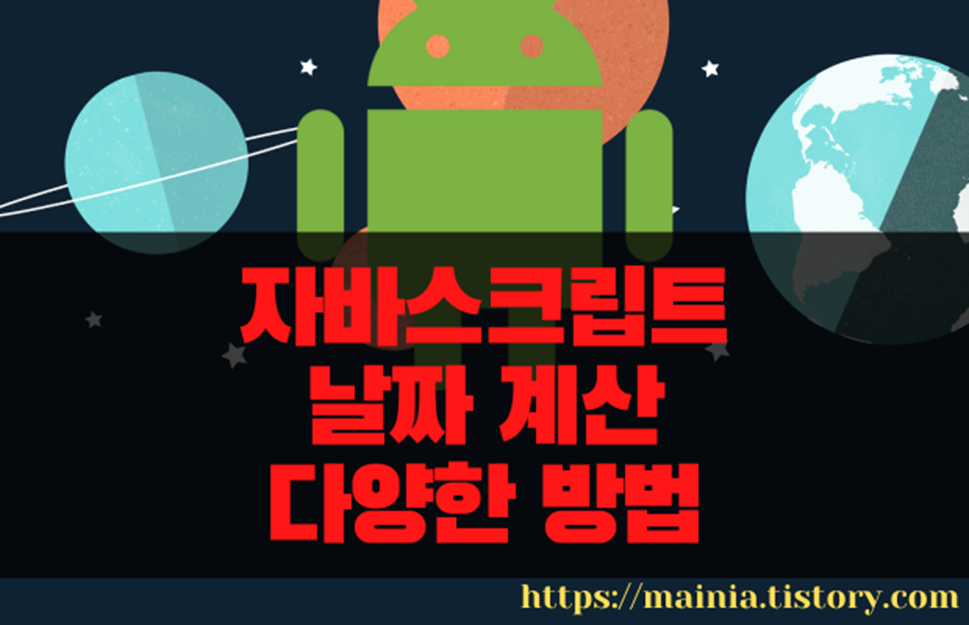
◎ 날짜 준비 |
▼ 먼저 계산을 위해 날짜를 준비했습니다. 텍스트로 날짜를 입력 받는 경우를 대비해 Date 객체로 변환하는 소스까지 들어가 있습니다. 년월일을 분리한 후 Date 의 생성자 함수에 인수로 각각 넣게 되면 해당 날짜의 Date 객체가 만들어 집니다.
var strDate1 = "2015-5-6";
var strDate2 = "2015-6-25";
var arr1 = strDate1.split('-');
var arr2 = strDate2.split('-');
var dat1 = new Date(arr1[0], arr1[1], arr1[2]);
var dat2 = new Date(arr2[0], arr2[1], arr2[2]);
◎ 일(day) 더하고 빼기 |
▼ 위에서 만든 두 개의 Date 객체에서 일을 더하고 빼려면 getDate() 함수로 일자를 구합니다. 일 값에 더하거나 빼고 나머지 년, 월은 getFullYear() 과 getMonth() 로 값을 구합니다. 그리고 문자열을 합쳐서 완성된 날짜를 만드는 것이죠.
// 날짜 더하고 빼기
document.write("* 현재날짜 : " + strDate1 + "<br/>");
document.write("* 3일 더하기 : " + dat1.getFullYear() + "-" + dat1.getMonth() + "-"
+ (dat1.getDate() + 3) + "<br/>");
document.write("* 3일 빼기 : " + dat1.getFullYear() + "-" +
dat1.getMonth() + "-" + (dat1.getDate() - 3) + "<br/><br/>");
◎ 월(Month) 더하고 빼기 |
▼ 일 더하기 빼기와 동일합니다. 단지 월을 구하기 위해 getMonth() 를 사용한 것만 다릅니다.
// 월 더하고 빼기
document.write("* 현재날짜 : " + strDate1 + "<br/>");
document.write("* 3개월 더하기 : " + dat1.getFullYear() + "-" +
(dat1.getMonth() + 3) + "-" + dat1.getDate() + "<br/>");
document.write("* 3개월 빼기 : " + dat1.getFullYear() + "-" +
(dat1.getMonth() - 3) + "-" + dat1.getDate()+ "<br/><br/>");
◎ 년(Year) 더하고 빼기 |
▼ 일 더하기 빼기와 동일하며 년도를 구하기 위해 getFullYear() 함수를 사용하였습니다. 그리고 완성된 날짜를 구성하기 위해 각 년월일을 문자열로 합쳤습니다.
// 년 더하고 빼기
document.write("* 현재날짜 : " + strDate1 + "<br/>");
document.write("* 3년 더하기 : " + (dat1.getFullYear() + 3) + "-" +
dat1.getMonth() + "-" + dat1.getDate() + "<br/>");
document.write("* 3년 빼기 : " + (dat1.getFullYear() - 3) + "-" +
dat1.getMonth() + "-" + dat1.getDate() + "<br/><br/>");
◎ 날짜 차이 알아내기 |
▼ 두 개의 Date 객체를 사칙연산으로 계산하게 되면 결과값으로 밀리세컨까지 연산된 숫자가 반환됩니다. 이 값으로 두 날짜 사이에 얼마나 차이가 나는지 알려면 24시, 60분, 60초, 1000값을 곱한 결과값으로 나누면 됩니다. 이렇게 나누게 되면 하루 단위를 구할 수 있습니다. 이것을 다시 30 으로 나누면 월이 구해 지고 30 * 12 로 나누면 년단위를 구할 수 있는 것이죠.
// 날짜 차이 알아 내기
var diff = dat2 - dat1;
var currDay = 24 * 60 * 60 * 1000;// 시 * 분 * 초 * 밀리세컨
var currMonth = currDay * 30;// 월 만듬
var currYear = currMonth * 12; // 년 만듬
document.write("* 날짜 두개 : " + strDate1 + ", " + strDate2 + "<br/>");
document.write("* 일수 차이 : " + parseInt(diff/currDay) + " 일<br/>");
document.write("* 월수 차이 : " + parseInt(diff/currMonth) + " 월<br/>");
document.write("* 년수 차이 : " + parseInt(diff/currYear) + " 년<br/><br/>");
◎ 또 다른 날짜 구하기 |
▼ 위에서 날짜를 구하고 뺄 때 년월일을 분리해서 문자열로 합치는 다소 번거로운 과정을 거쳤습니다. 아래 방법은 계산한 값을 년월일에 해당하는 함수에 셋팅 하면 간단하게 결과값을 얻을 수 있습니다. 출력할 때는 toLocaleString() 함수를 이용합니다.
// 또 다른 날짜 구하는 방법
dat1.setMonth(dat1.getMonth() + 3);
document.write("* 3개월 더하기 : " + dat1.toLocaleString() + "<br/>");
◎ 전체소스 |
▼ 위에서 구현한 전체 HTML 소스 입니다.
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=euc-kr">
<title>날짜 계산 </title>
<script type="text/javascript">
var strDate1 = "2015-5-6";
var strDate2 = "2015-6-25";
var arr1 = strDate1.split('-');
var arr2 = strDate2.split('-');
var dat1 = new Date(arr1[0], arr1[1], arr1[2]);
var dat2 = new Date(arr2[0], arr2[1], arr2[2]);
// 날짜 더하고 빼기
document.write("* 현재날짜 : " + strDate1 + "<br/>");
document.write("* 3일 더하기 : "
+ dat1.getFullYear() + "-" + dat1.getMonth() + "-" + (dat1.getDate() + 3)
+ "<br/>");
document.write("* 3일 빼기 : "
+ dat1.getFullYear() + "-" + dat1.getMonth() + "-" + (dat1.getDate() - 3)
+ "<br/><br/>");
// 월 더하고 빼기
document.write("* 현재날짜 : " + strDate1 + "<br/>");
document.write("* 3개월 더하기 : "
+ dat1.getFullYear() + "-" + (dat1.getMonth() + 3) + "-" + dat1.getDate()
+ "<br/>");
document.write("* 3개월 빼기 : "
+ dat1.getFullYear() + "-" + (dat1.getMonth() - 3) + "-" + dat1.getDate()
+ "<br/><br/>");
// 년 더하고 빼기
document.write("* 현재날짜 : " + strDate1 + "<br/>");
document.write("* 3년 더하기 : "
+ (dat1.getFullYear() + 3) + "-" + dat1.getMonth() + "-" + dat1.getDate()
+ "<br/>");
document.write("* 3년 빼기 : "
+ (dat1.getFullYear() - 3) + "-" + dat1.getMonth() + "-" + dat1.getDate()
+ "<br/><br/>");
// 날짜 차이 알아 내기
var diff = dat2 - dat1;
var currDay = 24 * 60 * 60 * 1000;// 시 * 분 * 초 * 밀리세컨
var currMonth = currDay * 30;// 월 만듬
var currYear = currMonth * 12; // 년 만듬
document.write("* 날짜 두개 : " + strDate1 + ", " + strDate2 + "<br/>");
document.write("* 일수 차이 : " + parseInt(diff/currDay) + " 일<br/>");
document.write("* 월수 차이 : " + parseInt(diff/currMonth) + " 월<br/>");
document.write("* 년수 차이 : " + parseInt(diff/currYear) + " 년<br/><br/>");
// 또 다른 날짜 구하는 방법
dat1.setMonth(dat1.getMonth() + 3);
document.write("* 3개월 더하기 : " + dat1.toLocaleString() + "<br/>");
</script>
</head>
<body>
</body>
</html>
※ 아래는 참고하면 좋을 만한 글들의 링크를 모아둔 것입니다. ※ ▶ 자바스크립트를 개발하기 위한 에디터 종류 ▶ 자바스크립트(JavaScript) 웹 소스 테스트 사이트 소개 ▶ 자바스크립트 escape, encodeURI, encodeURIComponent ▶ 자바스크립트 페이지 바로가기 기능 ▶ 자바스크립트 display 속성 이용해서 접거나 펴는 방법 |
'웹 프로그래밍 > 자바스크립트' 카테고리의 다른 글
자바스크립트(Javascript) 반복문 do while 구문 사용하기 (0) | 2023.11.19 |
---|---|
자바스크립트(javascript) 반복문 while 사용법 (1) | 2023.11.19 |
자바스크립트(Javascript) 반복문 사용하기 (0) | 2023.11.18 |
자바스크립트(Javascript) 반복문 for … of 구문 이용하기 (2) | 2023.11.14 |
자바스크립트(Javascript) for in 반복문 사용하기 (4) | 2023.11.13 |
자바스크립트 substring() 함수 이용해서 문자열 자르기 (2) | 2023.11.11 |
자바스크립트 indexof() 함수로 문자열 위치 찾기 (0) | 2023.11.11 |
자바스크립트 if, else if, else 조건문 사용해서 제어하기 (0) | 2023.11.11 |